Almost all apps you use today will run by accessing the internet. You can hardly find an app that runs without connecting to the internet. Internet has become inevitable part of our life as it solves one of the most critical problems of humans i.e., data transfer. You either receive or send a data to someone. Be it a social media app, news app or whatever type it may be, there’s a data transfer.
Hence it is super important to learn networking if you’re learning mobile app development. In this article I’ll be sharing about building a super simple mobile app that fetches the data from internet and render it on the app.
Table of Contents
How to Create the Project
Navigate to the folder where you want to create your project in the terminal and run the following command.
git clone https://github.com/5minslearn/Flutter-Boilerplate.git
Navigate to the Flutter-Boilerplate
folder and run the flutter pub get
command to install the dependencies.
cd Flutter-Boilerplate/
flutter pub get
That’s it. We’ve got our dependencies installed.
Open the project in Visual Studio Code by running the code ./
command in the terminal.
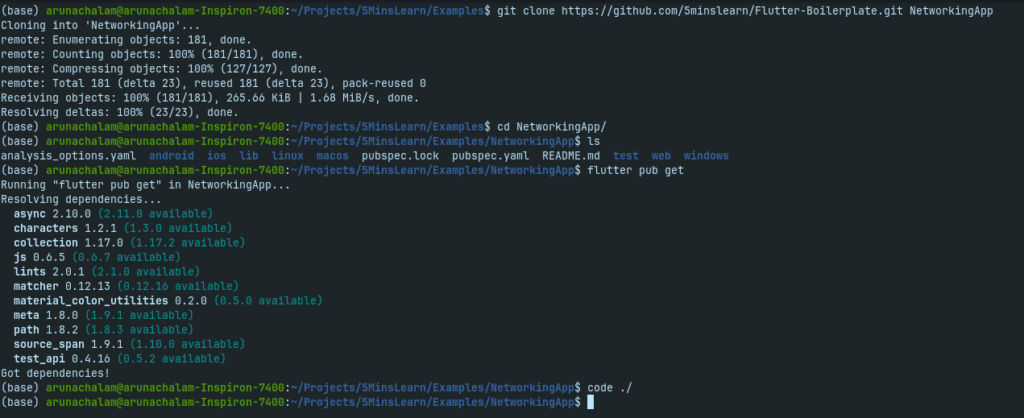
Start your emulator/connect your device and press F5
in VS Code to run your app.
At the moment, the app will just contain an empty screen as shown in the below screenshot.
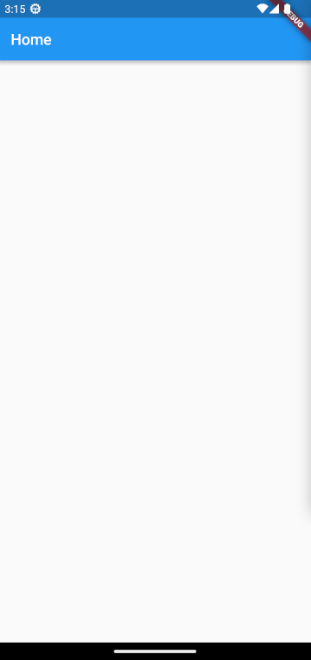
Let’s build our networking app.
Where to get the data
It is the most obvious question. If we were to fetch something from the internet and render, we need an API server exposing the data we need. But, most people cannot afford to do that for learning purpose. To overcome this many people are offering free API services.
You can consume the data from their API services for learning purpose. However, we cannot validate the originality of the data as most of them will be random.
In this blog, we’ll be using the API exposed by https://sampleapis.com/. They expose an API endpoint that lists the Coding Resources. The URL of the endpoint is https://api.sampleapis.com/codingresources/codingResources.
In our app, we’ll fetch the data from this endpoint and list them in our app.
Install the dependencies
Let’s install the dependencies needed to build this app. We need 2 dependencies. They are,
- http
- url_launcher
http
package is used to make a call to the API endpoint. The url_launcher
package is used to open a url in a external browser.
Open pubspec.yml
file and add the following two packages in the dependencies
section.
http: ^0.13.6
url_launcher: ^6.1.11
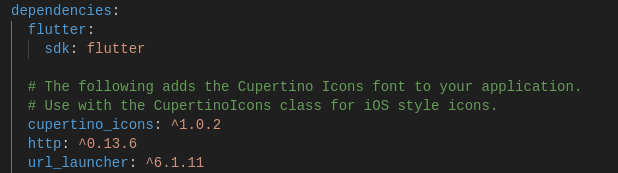
If you’re using VS Code as your IDE, the dependencies will be installed automatically by saving this file. For the other IDE’s, run flutter pub get
on your project root folder to install the dependencies.
Import the http
package in the lib/main.dart
file
import 'package:http/http.dart' as http;
Initialize a list object in the _MyHomePageState
class by adding the following code.
List _resources = [];
Let’s write a method that makes a call to our API endpoint and decode them into a JSON object.
void _fetchResources() async {
final response = await http.get(Uri.parse(
'https://api.sampleapis.com/codingresources/codingResources'));
if (response.statusCode == 200) {
final data = json.decode(response.body) as List;
setState(() {
_resources = data;
});
} else {
throw Exception('Failed to load resources');
}
}
Paste the above code into the _MyHomePageState
class. In the above method, we’re making a call to the API endpoint (https://api.sampleapis.com/codingresources/codingResources). From the response, we’re validating if it is successful in receiving the data by checking if it’s status code is 200
and throw error if it’s not. We then decode the received data and save it in our app’s state.
You may notice an error on pasting the above code at the json.decode
part. In order to decode a JSON, we need import a convert
package in our file. Add the following code at the top of the file.
import 'dart:convert';
Hopefully the error should be gone now.
We have a method that makes a call to the API endpoint and save the data in the state. Our next step is to trigger this method when we open the app.
We can do that by overriding the initState
method.
@override
void initState() {
super.initState();
_fetchResources();
}
From the Flutter documentation,
initState is called when this object is inserted into the tree. The framework will call this method exactly once for each State object it creates.
https://api.flutter.dev/flutter/widgets/State/initState.html
In the above code, we called our method _fetchResources()
in the initState()
method.
We got the list of items whenever we open the app. Our next step is to render them on UI.
Copy the below code and replace it with the build
method of the _MyHomePageState
class.
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: _resources.isEmpty
? const Center(
child: CircularProgressIndicator(),
)
: ListView.builder(
itemCount: _resources.length,
itemBuilder: (context, index) {
final resource = _resources[index];
return InkWell(
onTap: () => {},
child: Card(
child: ListTile(
title: Text(resource['description']),
subtitle: Text(resource['url']),
leading: const CircleAvatar(
backgroundImage: NetworkImage(
"https://images.unsplash.com/photo-1547721064-da6cfb341d50")),
trailing: Text(resource['types'].join(', ')),
)));
}));
}
Let’s understand the above code.
We show a loader if our state has empty values. If it has some values in it, we’ll iterate through as a ListView builder and we render a Card widget for each item, displaying the description
, url
, and types
of the resource.
That’s it.
Run the app by pressing F5
and you should be able to see the following screenshot.
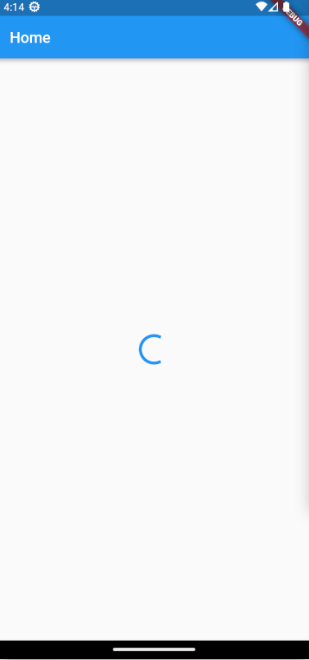
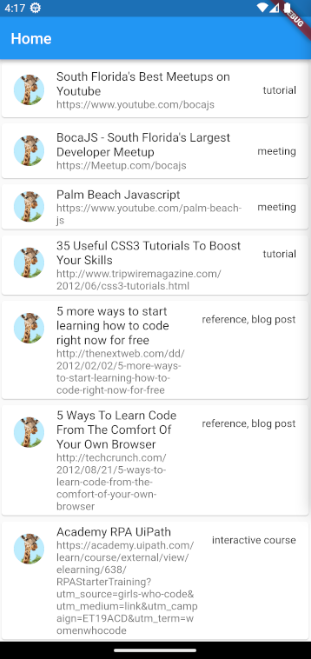
That’s Awesome!!! Isn’t it?
But I feel there’s one small thing that’s missing in this. Briefing it here.
We’re able to see the list of the resources. But, we’re not able to view those resource. Some resources has small link that we can easily remember and type. But, few of them have a long url which is not possible for a typical human being to remember. Let’s add a small enhancement that when we press a resource, it’s link should be opened in our default browser.
The implementation of it is so simple in Flutter. This is the reason we added url_launcher
package at the beginning of this blog.
Import the url_launcher
package in our app.
import 'package:url_launcher/url_launcher.dart';
Add the following method in the _MyHomePageState
class.
_launchURL(String url) async {
if (await canLaunch(url)) {
await launch(url);
} else {
throw 'Could not launch $url';
}
}
The above method accepts a url and validates the link and opens it in the browser.
We have to call this method on tapping the card. We can achieve that by calling this method in the onTap
property of the InkWell
widget.
Here’s the code for it.
onTap: () => {_launchURL(resource['url'])},
Let’s run our app and test this.
Hope you were disappointed on tapping a card. Yes. I’m also disappointed while working on this.
You would have faced this error.
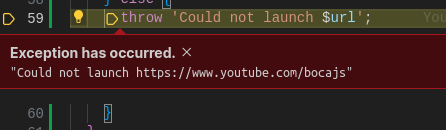
Though the url is right, why is our system not opening this in a browser.
Well. We have to learn about intent action.
Quoting it from Android Developers documentation,
An Intent provides a facility for performing late runtime binding between the code in different applications. Its most significant use is in the launching of activities, where it can be thought of as the glue between activities. It is basically a passive data structure holding an abstract description of an action to be performed.
https://developer.android.com/reference/android/content/Intent
This basically means when we hand over something to the external app, we have to declare that in our app. For Android we have to define it in AndroidManifest.xml
and for iOS most of these configurations go into Info.plist
file.
Add the queries
block in the following code to your AndroidManifest.xml
file.
<manifest>
<application>
...
</application>
<queries>
<intent>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="https" />
</intent>
</queries>
</manifest>
Uninstall the app from your mobile and run the app again.
Hopefully you should be able to see the link opened in the browser.
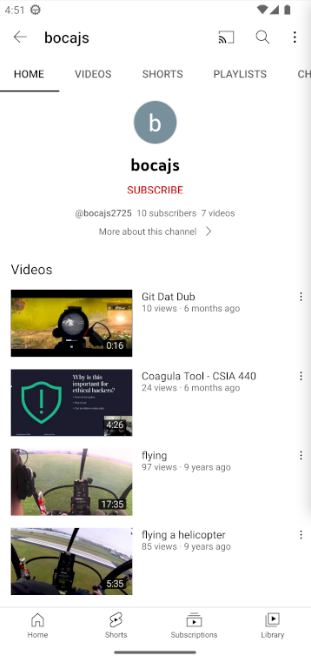
Conclusion
In this article, you’ve learnt about networking in Flutter. We made a request to an API, render the list, and open the url in the browser.
This repo has my code. You can use it for your reference.
If you wish to learn more about Flutter, subscribe to my article by entering your email address in the below box.
Have a look at my site which has a consolidated list of all my blogs.