Nowadays, We can see most people using software with the logo of a whale lifting some squares ( containers ). Yes, I am talking about the docker. Actually, the Docker logo symbolizes software that brings together a huge amount of organized information. This is a hint of convenience and ease of work.
Most enterprises adapted to Docker-based deployments (also called containerized deployments) nowadays. This blog will be the best fit for those who know nothing apart from the term “Docker”.
In this article, you’ll learn about the fundamentals of Docker, build your own Docker image and publish it to the Docker Hub.
Let’s understand why we need docker with a simple example.
Let’s assume you’re joining a new company and you have been allocated to an extremely huge project to work on. You received a brand new laptop and are ready to put on your development shoes. The first step whenever you are onboarded into a project is to set up the development environment. Since it’s an extremely huge enterprise project, it consumes a bunch of time to set up the development environment. You may need to install project-specific dependencies, tools, and many more. In the middle, you may face errors that you can mostly solve by following the README guide, but a couple of them might happen for the first time (might happen due to laptop configuration) and you have to solve them on your own.
Imagine if you have to follow the same process for large team members. It’ll be horrible to handle right?
This is where docker comes really handy. Docker will create containerized applications that run on any type of environment and It has all the dependencies within it. Docker solves many use cases, the above scenario is one among them.
By using docker we can standardize application operations, ship code faster, and seamlessly deploy to Production.
Table of Contents
How does Docker work?
Docker provides a standardized way to develop, test and deploy code.
Docker can be imagined as a super-powered advanced virtual machine. Let’s learn it with an example.
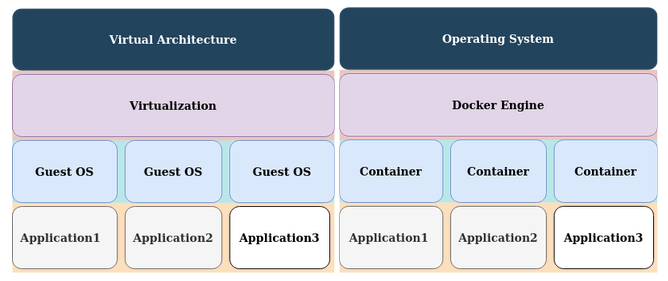
Before getting started to work on docker, we should understand some fundamentals of it. They are,
- Docker Engine
- Docker Container
- Docker Image
What is Docker Engine?
The Docker engine is an open-source containerization technology to build a containerizing application. We can use the docker engine on a variety of platforms through Docker Desktop or Binary Installation. To simplify, the software that hosts the containers is called Docker Engine.
What is Docker Container?
A container is a standard unit of software that packages up the code and all required dependencies to run an application on different platforms or computing environments.
Containers are fully isolated from the computing environment, so applications run quickly and reliably from one computing environment to another.
Docker containers are built from container images.
What is a Docker image?
A Docker container image is a lightweight, standalone, executable package of software that includes everything needed to run an application. It includes code, runtime, system tools, system libraries, and settings.
As we discussed, Containers are built from images. Images become containers when the it starts running on a Docker Engine.
Why should I use docker?
Docker gives the ability to run an application in any type of environment which is completely isolated from the current environment, and that is called a container. This isolation and security allow us to run as many containers in a host. Docker helps developers to work in a standardized environment using local containers which provide all the packages and dependencies to run an application.
Let’s see the use cases of docker,
- Developers can write code locally and share their work using Docker Containers
- We can use the docker to push our applications into a test/production environment and execute automated and manual tests
- When developers need to fix something, they can easily make the changes and push the docker image to the testing or production environment
Just like git, we can use docker, If we make any changes we can just push the docker images and pull them into the host machine, No changes need to be done in the host server.
Here are the ideal deployment steps before the arrival of Docker,
- Pull/clone the code from the Git
- Install dependencies, Run migrations, etc. in the host machine.
- Start the application
We will repeat the steps on every server whether it is a testing/production environment.
Here is the deployment steps using Docker
- Pull the docker image
- Run the container in the host machine
What is Docker Hub?
Docker Hub is the largest community for the docker images. It allows us to share the container images among the team, or the Docker community. Imagine Docker Hub as a similar version of GitHub. Here, Docker images reside instead of project’s code.
We can pull the open-source docker images also. To use this we have to create an account in the docker hub.
How to Containerize Nodejs Application using Docker?
Prerequisites:
- A Basic understanding of Nodejs Applications
- Docker desktop application
You can install the Docker Desktop application by following their original documentation.
You can verify if docker is installed on your machine by querying it’s version,
docker -v
It is preferrable to have Node version 14 and above. You can quickly check that by running the below command,
node -v
Let’s start our implementation.
Instead of starting over from scratch, I have created a super simple Express API that exposes only one endpoint. I pushed the code to a public repo on Github. Clone the repo by running the below command.
git clone https://github.com/5minslearn/node_with_docker
This is the project’s structure.
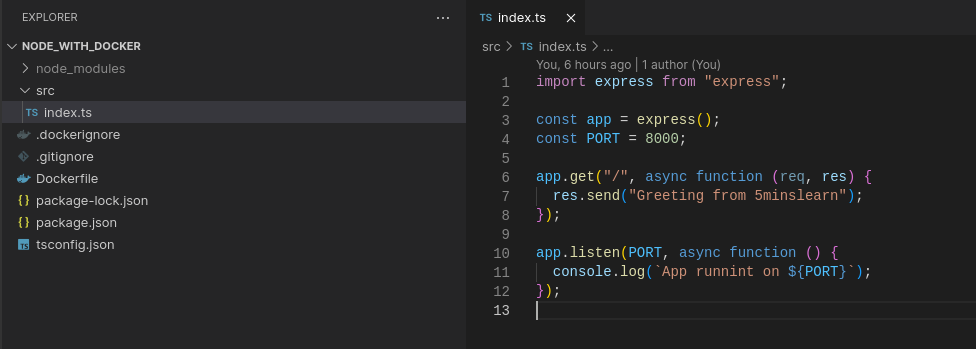
I have created only one endpoint (“/”) and calling it will return “Greeting from 5minslearn”. I’ve simplified as much as possible to focus more on docker.
Create docker file
You have to create a file named “Dockerfile” in the root directory. It is the default file name for the docker engine. Paste the following code into the file.
FROM node:latest
WORKDIR /app
COPY . /app
RUN npm install
EXPOSE 8000
CMD ["npm","start"]
Let’s understand these command line by line.
Defining the parent image is the first step of the docker engine. You have to define the parent image from which you’re building the project. In our case it’s Node. There are a lot of parent images available in Docker Hub. You have to define the Image Variant next to the parent image. I always prefer to use the latest node image.
FROM node:latest
Second step is to define the working directory in docker. Let’s define our working directory as app
directory.
WORKDIR /app
Copy the project to the app
directory, Ensure to exclude the node_modules
directory. We will see how to ignore file/folder in the upcoming steps.
COPY . /app
In the above command, the .
indicates that all the files and directories are to be copied to the app
folder.
Next step is to install the required dependencies.
RUN npm install
RUN is an image build step. The state of the container after a RUN command will be committed to the container image. A Dockerfile can have many RUN steps that can layer on top of one another to build the image.
Expose the port in which application should run.
EXPOSE 8000
The EXPOSE instruction informs Docker that the container listens on the specified network ports at runtime.
Finally run the execution command.
CMD ts-node src/index.ts
CMD is the command the container executes by default when we launch the built image.
Tips:
I recommend you to install Docker
extension if you’re using VS Code. It’ll help you with it’s solid suggestions.
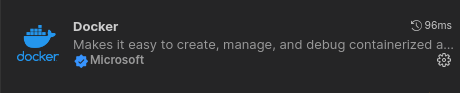
Docker
extensionHow to ignore files to be copied into a docker container
You have to exclude the unwanted files to be copied to the container. .dockerignore
file helps you with that. It works like .gitignore
for Git.
You can define all the files you have to ignore from copying.
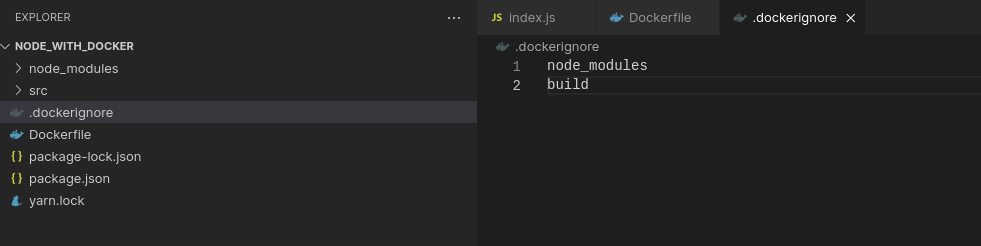
Great! You completed the docker configuration. Let’s run the application.
How to build docker images
Docker images can be built by running docker build
command. Here’s the syntax for it.
docker build -t image_name:version_number .
image_name
indicates the container image name and version_number
indicates the image version. Hope you notice the dot (.
) at the end of the command. It indicates that the docker image should be built from the current directory.
I’m entering a random name for my image as aanandggs/node_with_docker
. You may choose to enter whatever you want.
docker build -t aanandggs/node_with_docker:0.0.1 .
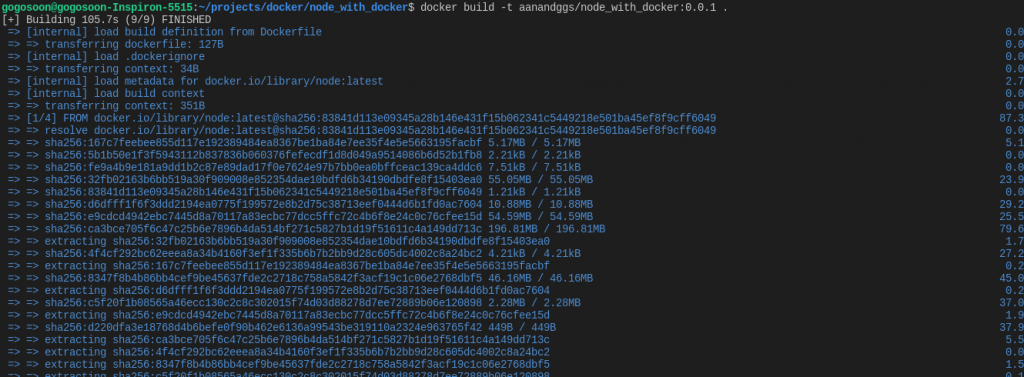
Once building your Docker image, you’ll be able to see it on your Docker Desktop.
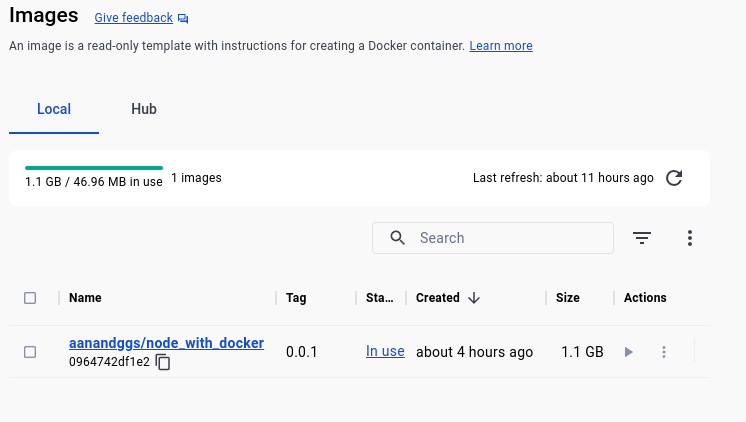
How to run the docker image
As we build the docker image, our next step is to run the docker image. When an image started running on a Docker Engine, it’ll become a container.
Let’s run our image which we built in the previous step.
docker container run -d --name -p : :`image
docker container run -d --name docker_with_node -p 8000:8000 aanandggs/node_with_docker:0.0.1

Let’s understand the above command.
docker container run
command is used to create and run a new container from an image
-d
flag instructs the container to run on the background (detach). It prints the container id.
--name
parameter is used to give a name to our container
-p
parameter is used to publish a container’s port to the host. It’ll bind the port 8000
of your local machine with the port 8000
of the docker container
image_name:version
indicates the image and it’s version that this container should run
You can have a look at the running containers in the Docker Desktop app.
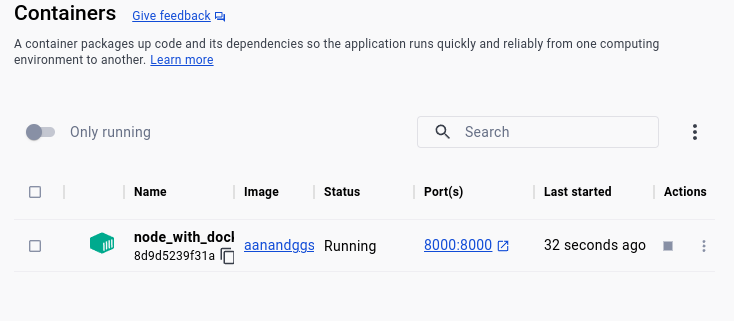
Our app is running. Let’s check the output on the browser by trying to access the “/” endpoint. Hit locahost:8000/
on your browser. You should see a message similar to the one in the below screenshot.
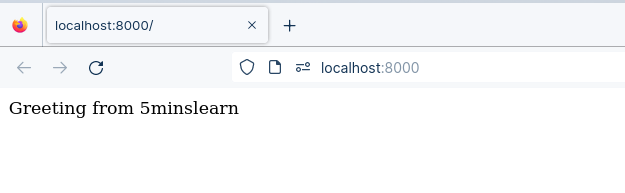
You have successfully ran a app with the docker container.
Note:
You can bind any local port to the docker port. Ensure your local port is not used by any other process.
How to push the image to the DockerHub
Create your profile on Docker Hub. Come back to terminal and run the below command to login to Docker CLI.
docker login
If you face any issues with login, Please follow this doc to login to the Dockerhub on your machine.
After the successful login, We can push the image to the docker hub.
docker push :
docker push aanandggs/node_with_docker:0.0.1
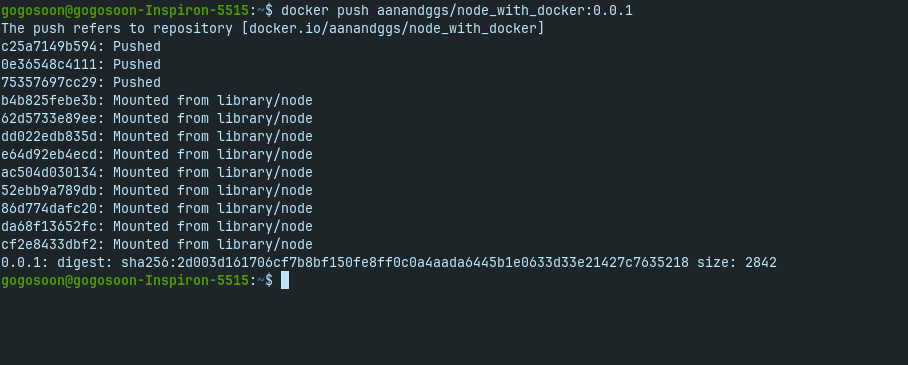
Hurray!!! Images are uploaded to the docker hub.
You’ll be able to see them in the docker hub console.
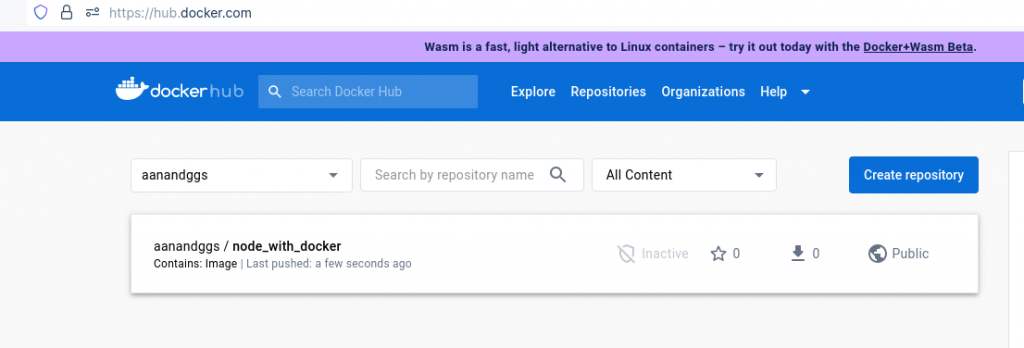
Since our image is public, anyone on the internet can pull the image and run it on their machines without any third-party installation.
Conclusion
Hope you folks enjoyed reading this blog. Will see you in another interesting blog. Do comment with your thoughts. If you have any queries or corrections regarding this blog, feel free to reach out.
If you wish to learn more about Docker, subscribe to my article by entering your email address in the below box.
Have a look at my site which has a consolidated list of all my blogs
Cheers !!!